This article discusses the use of 4 timers of the ESP32 to be used to run the program when it meets the specified time. Difference Between Using Timer and Delay time.sleep()/time.sleep_ms()/time.sleep_us() is that time delay is a loop to allow the processor to spend time looping to complete the specified amount of time whereas the timer uses the principle of having a function run every time a specified time is reached, so while a timer does not call a function to execute the processor has idle time for other processing, and unlike interrupts, it is a timer interrupt instead of a pin state interrupt connected to an external circuit.
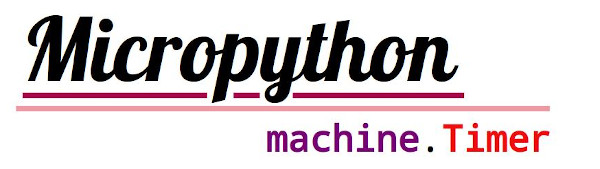
Timer
ESP32 timers or timers have two groups, each containing two sub-hardware timers, four of which are 64-bit timers running 16-bit pre-scalars and 64-bit up/down counters supporting automatic reloading
Calling the timer class for the ESP32 microcontroller is done by calling the Timer class under the machine class as follows:
from machine import Timer
The creation of a timer object has the following form of declaration, timer number is the sequence number of the timer to be used which are the values 0, 1, 2, and 3.
object = Timer( timer_number )
When there is a timer object available, configure the timer behavior with the init( ) command as follows.
object.init( period, mode, callback )
where
- period is the operating time of the timer. It is in milliseconds.
- mode is a timer working mode that has 2 working modes as follows.
- Timer.ONE_SHOT for being executed only once.
- Timer.PERIODIC for calling the callback function every specified period.
- callback is the function that is called when the timer runs.
To cancel holding the timer can be done with the following command.
object.deinit( )
Example code demoTimer0.py allows the speaker to emit a sound once after a set time of 2 seconds by connecting the speaker to the GPIO25 pin. The program will emit a beep even with a 5 seconds delay.
###########################################
# demoTimer0.py
# (C) 2021, JarutEx
# https://www.jarutex.com
###########################################
from machine import Timer
from machine import Pin
from time import sleep_ms
def beep(x):
spkPin = Pin( 25, Pin.OUT )
spkPin.on()
sleep_ms(50)
spkPin.off()
try:
tmr0 = Timer(0)
except:
print("cannot construct Timer(0)!")
try:
tmr0.init( period=2000, mode=Timer.ONE_SHOT, callback=beep)
except:
print("cannot init()!")
sleep_ms(5000)
if (tmr0):
tmr0.deinit()
Example code demoTimer1.py changes the vocalization pattern to occur every 2 seconds by setting the mode to Timer.PERIODIC and the program has 2 beeps, the first after 2 seconds, the second when entering the 4th second, after 1 second it is the end of the 5 seconds delay and the program ends.
###########################################
# demoTimer1.py
# (C) 2021, JarutEx
# https://www.jarutex.com
###########################################
from machine import Timer
from machine import Pin
from time import sleep_ms
def beep(x):
spkPin = Pin( 25, Pin.OUT )
spkPin.on()
sleep_ms(50)
spkPin.off()
try:
tmr0 = Timer(0)
except:
print("cannot construct Timer(0)!")
try:
tmr0.init( period=2000, mode=Timer.PERIODIC, callback=beep)
except:
print("cannot init()!")
sleep_ms(5000)
if (tmr0):
tmr0.deinit()
Conclusion
From this article, you will find that implementing a timer can be set to run the function at a specific time or run at a preset time. This is considered a type of interrupt but from a source of four available timers allowing programmers to use the chip more efficiently. Finally, have fun with programming.
References
- Espressif. “General Purpose Timer”
- Micropython, “Quick Reference for ESP8266”
- Micropython. “Quick Reference for ESP32”
- Micropython, “class Timer – control hardware timers”
(C) 2020-2021, By Jarut Busarathid and Danai Jedsadasthitikul
Updated 2021-10-23