This article compiles information about operators used in C++ to write expressions of commands. Operator marks are divided into five groups: Arithmetic, Boolean, Comparison, bitwise, and Compound.
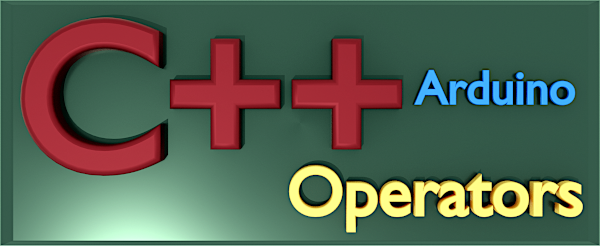
Arithmetic operator
The arithmetic operator is computational mark. The form of use is in the following format.
LOpr Operator ROpr
The operator list is as follow.
Name | mark | description |
defining | = | bring ROpr store in LOpr |
addition | + | Return addition from LOpr with ROpr |
Subtraction | – | Return subtraction from LOpr with ROpr |
multiplication | * | Return multiplication from LOpr with ROpr |
division | / | Return division from LOpr with ROpr |
modulo division | % | Return modulo division from LOpr with ROpr |
Boolean operator
A boolean operator is a logical comparison, in C++ treats false as 0, whereas true is any value other than 0. The logical action consists of and, or, and negation, as shown in the following table.
name | operator | usage | description |
and | && | LOpr && ROpr | if LOpr nad ROpr are true, return true otherwise false |
or | || | LOpr || ROpr | if LOpr or ROpr is true return true except only if LOpr and ROpr are false |
not | ! | ! Operand | if Operand is true return false otherwise true |
Comparison operator
A comparison operator is about comparing the left value with the right value and return the answer to true or false. The form of usage is in the following format.
LOpr Operator ROpr
The operator list is as follows.
name | operator | description |
equal | == | return true if LOpr is equal to ROpr |
not equal | != | return true if LOpr is not equal to ROpr |
less than | < | return true if LOpr is less than ROpr |
greater than | > | return true if LOpr is greater than ROpr |
less than or equal | <= | return true if LOpr is less than or equal to ROpr |
greater than or equal | >= | return true if LOpr is greater than or equal to ROpr |
Bitwise operator
เครื่องหมายดำเนินการทางบิตมีความแตกต่างจากการดำเนินการแบบบูลตรงที่ การดำเนินการแบบบูลนั้นพิจารณาค่าของตัวดำเนินการ ถ้ามีค่าเป็น 00 หมายถึงเป็น false และถ้าไม่ใช่ 0 มองค่านั้นเป็น true แต่กรณีของการดำเนินการทางบิตนั้นเป็นการกระทำในระดับบิต โดยเทียบบิตของแต่ละหลักของตัวดำเนินการทางซ้ายและทางขวาตามคุณสมบัติของการเปรียบเทียบเพื่อสร้างบิตผลลัพธ์ของบิตในหลักนั้นออกมา ซึ่งเครื่องหมายที่ใช้ในกลุ่มนี้คือ การและ การหรือ การเอ็กคลูซีฟออร์ และการกลับบิต
Bit-LOpr | Bit-ROpr | Bit-LOpr & Bit-ROpr |
---|---|---|
0 | 0 | 0 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
Bit-LOpr | Bit-ROpr | Bit-LOpr | Bit-ROpr |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 1 |
Bit-LOpr | Bit-ROpr | Bit-LOpr ^ Bit-ROpr |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
Bit-Operand | ~ Bit-Operand |
---|---|
0 | 1 |
1 | 0 |
In addition to the above actions, there are two other bit operations: shift left and shift right. The form of use is as follows.
Operand << The number of bits to be shifted to the left.
Operand >> The number of bits to be shifted to the right.
Compound operator
The compound operator combines the workflow steps into a single command. The operator in this group are as follows:
name | operator | usage | description |
increment | ++ | operand++ | 1. Apply the Operand value. 2. Operand = Operand+1 |
increment | ++ | ++operand | 1. Operand = Operand+1 2. Apply the Operand value. |
decreasement | — | operand— | 1. Apply the Operand value. 2. Operand = Operand-1 |
decreasement | — | —operand | 1. Operand = Operand-1 2. Apply the Operand value. |
addition | += | LOpr += ROpr | LOpr = LOpr + ROpr |
subtraction | -= | LOpr -= ROpr | LOpr = LOpr – ROpr |
multiplication | *= | LOpr *= ROpr | LOpr = LOpr * ROpr |
division | /= | LOpr /= ROpr | LOpr = LOpr / ROpr |
modulo division | %= | LOpr %= ROpr | LOpr = LOpr % ROpr |
and | &= | LOpr &= ROpr | LOpr = LOpr & ROpr |
or | |= | LOpr |= ROpr | LOpr = LOpr | ROpr |
Conclusion
From this article, you have already been familiar with the various operator marks in the C++ language of Arduino. In the next article, we will learn how to create expressions. Finally, have fun with programming.
Reference
(C ) 2020-2021, By Jarut Busarathid and Danai Jedsadathitikul
Updated 2021-09-26